D.1 The OutputDirectory
Class and KSL
Object
We often need to get data into a program or to write data out. Because of the plethora of file formats and differing methods for creating and using files, the KSL provides some utilities for working with comma separated value (CSV), Excel, Markdown, text, and database files. The most relevant classes include OutputDirectory
class, the KSL
object and the KSLFileUtil
class.
Figure D.1 illustrates the functions and properties of the KSL
object and the OutputDirectory
class.
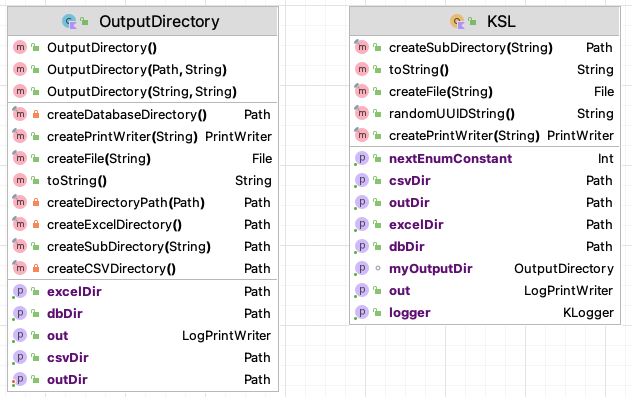
Figure D.1: OutputDirectory and KSL
The OutputDirectory
class is an abstraction for a file directory to store output. When working with a particular simulation model, it is useful to store all of the results and files generated by the model in one directory. The OutputDirectory
class facilitates this common use case. An instance of OutputDirectory
requires a path to the file directory and then forms some standard sub-directories (excelDir
, dbDir
, csvDir
, outDir
) to hold various files that may be generated for these common types of files. The KSL
object is, in essence, a default directory to hold all KSL output. Notice that OutputDirectory
provides functions for creating files and directories within it. The most useful of the functions is the createPrintWriter(name: String)
function which takes in the name of the file and creates a PrintWriter
set up to write to the file. Also, notice the createSubDirectory()
function. The directories created by this function are relative to the defined output directory. This alleviates the burden of fully specifying path strings and working with paths.
Here is some sample code that uses the OutputDirectory
class.
// get the working directory
val path = Paths.get("").toAbsolutePath()
println("Working Directory = $path")
// creates a directory called TestOutputDir in the current working directory
// Creates subdirectories: csvDir, dbDir, excelDir and file out.txt
val outDir = OutputDirectory(path.resolve("TestOutputDir"))
// write to the default file
outDir.out.println("Use out property to write out text to a file.")
// Creates a PrintWriter (and file) to write to within TestOutputDir
val pw = outDir.createPrintWriter("PW_File.txt")
pw.println("Hello, World")
val subDir = outDir.createSubDirectory("SubDir")
println(outDir)
The KSL
object is essentially a predefined instance of OutputDirectory
that creates a default directory called kslOutput
within the current working directory. The property out
is an instance of a LogPrintWriter
, which is a class that wraps a PrintWriter
but also has a property called OUTPUT_ON
, which has type boolean, where true indicates that output will be written and false turns off the output. You can use this to stop excessive print messages globally. I find this useful for simple debugging messages. Besides this field, KSL
has a standard logger for logging program messages.
// use KSL like you use OutputDirectory but with some added functionality
// The PW_File.txt file with be within the kslOutput directory within the working directory
val pw = KSL.createPrintWriter("PW_File.txt")
pw.println("Hello, World!")
// Creates subdirectory SubDir within the kslOutput directory
KSL.createSubDirectory("SubDir")
// use KSL.out to write to kslOutput.txt
KSL.out.println("Information written into kslOutput.txt")
println(KSL)
// KSL also has logger. This logs to logs/ksl.log
KSL.logger.info { "This is an informational log comment!" }