D.7 Array Utilities
The KSL provides a wide-variety of functions and extensions for working with Double
, Int
, and Long
arrays. Most of the functionality is focused on working with one-dimensional Double
arrays.
Figure D.13 illustrates the many methods for working with arrays via the KSLArrays
object. These methods are also available as extension functions.
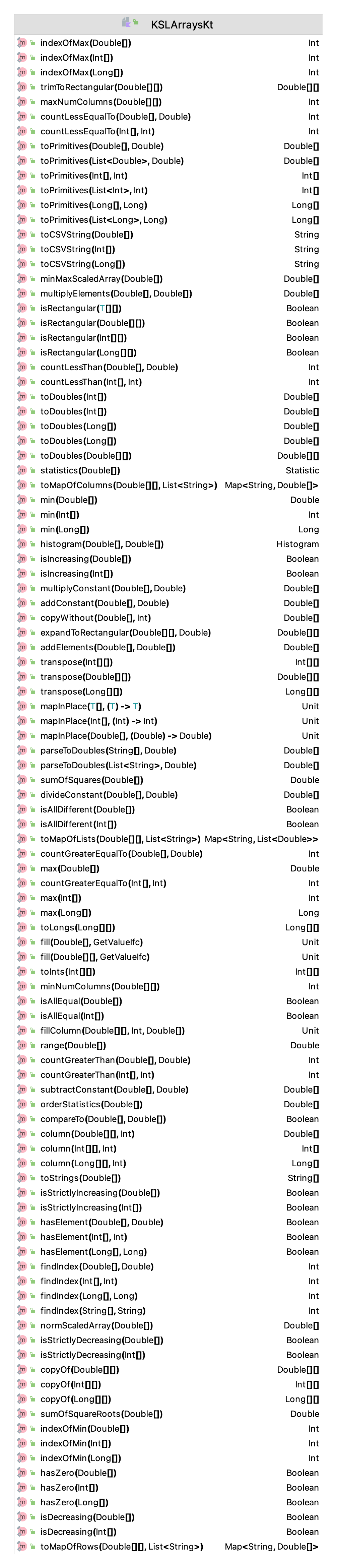
Figure D.13: Array Utilities
There are a number of functions that facilitate conversions: to primitive types, to strings, conversion between types, and parsing.
- toPrimitives(Double[], Double) Double[]
- toPrimitives(List\(<\)Double\(>\), Double) Double[]
- toPrimitives(Int[], Int) Int[]
- toPrimitives(List\(<\)Int\(>\), Int) Int[]
- toPrimitives(Long[], Long) Long[]
- toPrimitives(List\(<\)Long\(>\), Long) Long[]
- toCSVString(Double[]) String
- toCSVString(Int[]) String
- toCSVString(Long[]) String
- toDoubles(Int[]) Double[]
- toDoubles(Int[]) Double[]
- toDoubles(Long[]) Double[]
- toDoubles(Long[]) Double[]
- toDoubles(Double[][]) Double[][]
- parseToDoubles(String[], Double) Double[]
- parseToDoubles(List\(<\)String\(>\), Double) Double[]
- toStrings(Double[]) String[]
- toInts(Int[][]) Int[][]
- toLongs(Long[][]) Long[][]
Then, there are useful functions for performing basic statistical computations such as finding the indices of the minimum or maximum, counting elements, finding the minimum, finding the maximum, and computing statistics.
- indexOfMax(Double[]) Int
- indexOfMax(Int[]) Int
- indexOfMax(Long[]) Int
- indexOfMin(Double[]) Int
- indexOfMin(Int[]) Int
- indexOfMin(Long[]) Int
- countLessEqualTo(Double[], Double) Int
- countLessEqualTo(Int[], Int) Int
- countLessThan(Double[], Double) Int
- countLessThan(Int[], Int) Int
- statistics(Double[]) Statistic
- min(Double[]) Double
- min(Int[]) Int
- min(Long[]) Long
- histogram(Double[], Double[]) Histogram
- countGreaterEqualTo(Double[], Double) Int
- max(Double[]) Double
- countGreaterEqualTo(Int[], Int) Int
- max(Int[]) Int
- max(Long[]) Long
- range(Double[]) Double
- countGreaterThan(Double[], Double) Int
- countGreaterThan(Int[], Int) Int
- subtractConstant(Double[], Double) Double[]
- orderStatistics(Double[]) Double[]
- sumOfSquares(Double[]) Double
- sumOfSquareRoots(Double[]) Double
In addition, there are methods for checking the arrays. For example, methods exist to check if the 2-D array is rectangular in shape. That is, the rows all have the same number of elements. You can also check if the elements are all different, are strictly increasing or decreasing, and contain a zero value.
- isRectangular(T[][]) Boolean
- isRectangular(Double[][]) Boolean
- isRectangular(Int[][]) Boolean
- isRectangular(Long[][]) Boolean
- isIncreasing(Double[]) Boolean
- isIncreasing(Int[]) Boolean
- isAllDifferent(Double[]) Boolean
- isAllDifferent(Int[]) Boolean
- isStrictlyIncreasing(Double[]) Boolean
- isStrictlyIncreasing(Int[]) Boolean
- hasElement(Double[], Double) Boolean
- hasElement(Int[], Int) Boolean
- hasElement(Long[], Long) Boolean
- findIndex(Double[], Double) Int
- findIndex(Int[], Int) Int
- findIndex(Long[], Long) Int
- findIndex(String[], String) Int
- isStrictlyDecreasing(Double[]) Boolean
- isStrictlyDecreasing(Int[]) Boolean
- hasZero(Double[]) Boolean
- hasZero(Int[]) Boolean
- hasZero(Long[]) Boolean
- isDecreasing(Double[]) Boolean
- isDecreasing(Int[]) Boolean
Then, there are methods for operating on the entire array. First, there are functions to reshape the arrays such as trimming non-rectangular arrays to rectangular, transposing the arrays, and expanding non-rectangular arrays to be rectangular. Then, there are functions to apply to each element such as multiplying the elements by a constant, adding a constant, and dividing by a constant. You can also apply a function to the elements in-place. That is transform each element without creating a new array.
- trimToRectangular(Double[][]) Double[][]
- minMaxScaledArray(Double[]) Double[]
- multiplyElements(Double[], Double[]) Double[]
- multiplyConstant(Double[], Double) Double[]
- addConstant(Double[], Double) Double[]
- copyWithout(Double[], Int) Double[]
- expandToRectangular(Double[][], Double) Double[][]
- transpose(Int[][]) Int[][]
- transpose(Double[][]) Double[][]
- transpose(Long[][]) Long[][]
- divideConstant(Double[], Double) Double[]
- normScaledArray(Double[]) Double[]
- toMapOfLists(Double[][], List\(<\)String\(>\)) Map\(<\)String, List\(<\)Double\(>\)\(>\)
- toMapOfColumns(Double[][], List\(<\)String\(>\)) Map\(<\)String, Double[]\(>\)
- toMapOfRows(Double[][], List\(<\)String\(>\)) Map\(<\)String, Double[]\(>\)
- mapInPlace(T[], (T) -\(>\) T) Unit
- mapInPlace(Int[], (Int) -\(>\) Int) Unit
- mapInPlace(Double[], (Double) -\(>\) Double) Unit
- fillColumn(Double[][], Int, Double[]) Unit
- fill(Double[], GetValueIfc) Unit
- fill(Double[][], GetValueIfc) Unit
Figure D.14 illustrates the many methods for permuting and sampling arrays.
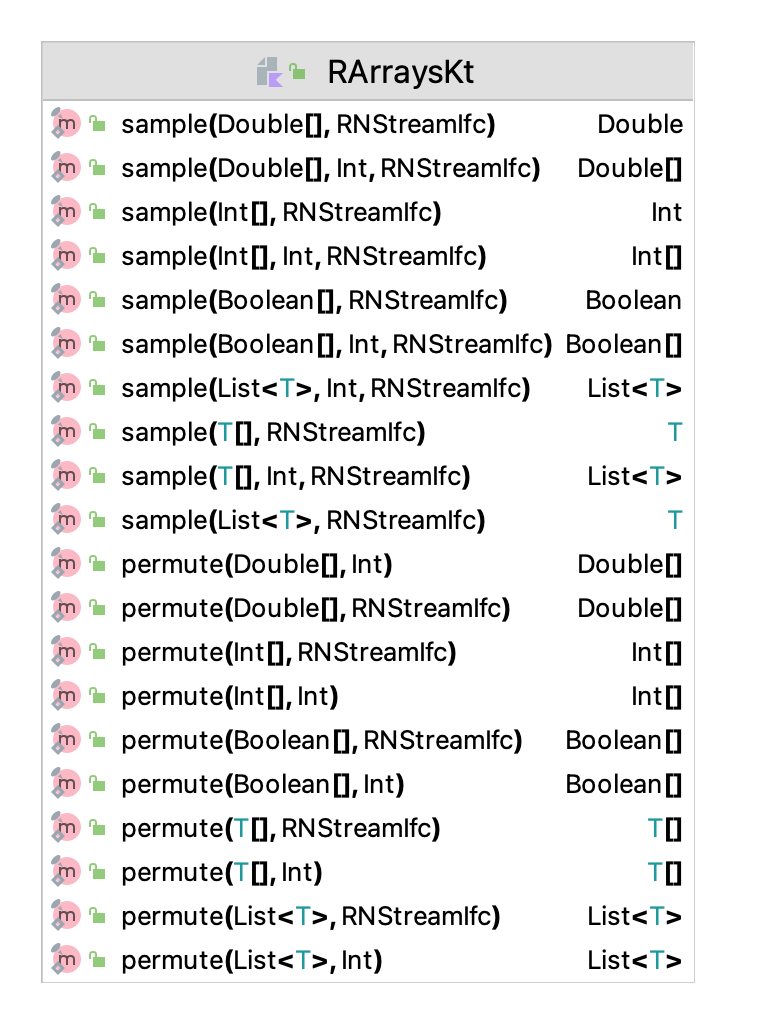
Figure D.14: Random Array Utilities
Much of the functionality described here can (and has been) used to implement more complex operations used within the KSL. There are other libraries that are under active develop for working with arrays with design goals that focus on efficiency and memory. The interested reader might look at kmath and the multi-dimensional arrays library, multik.